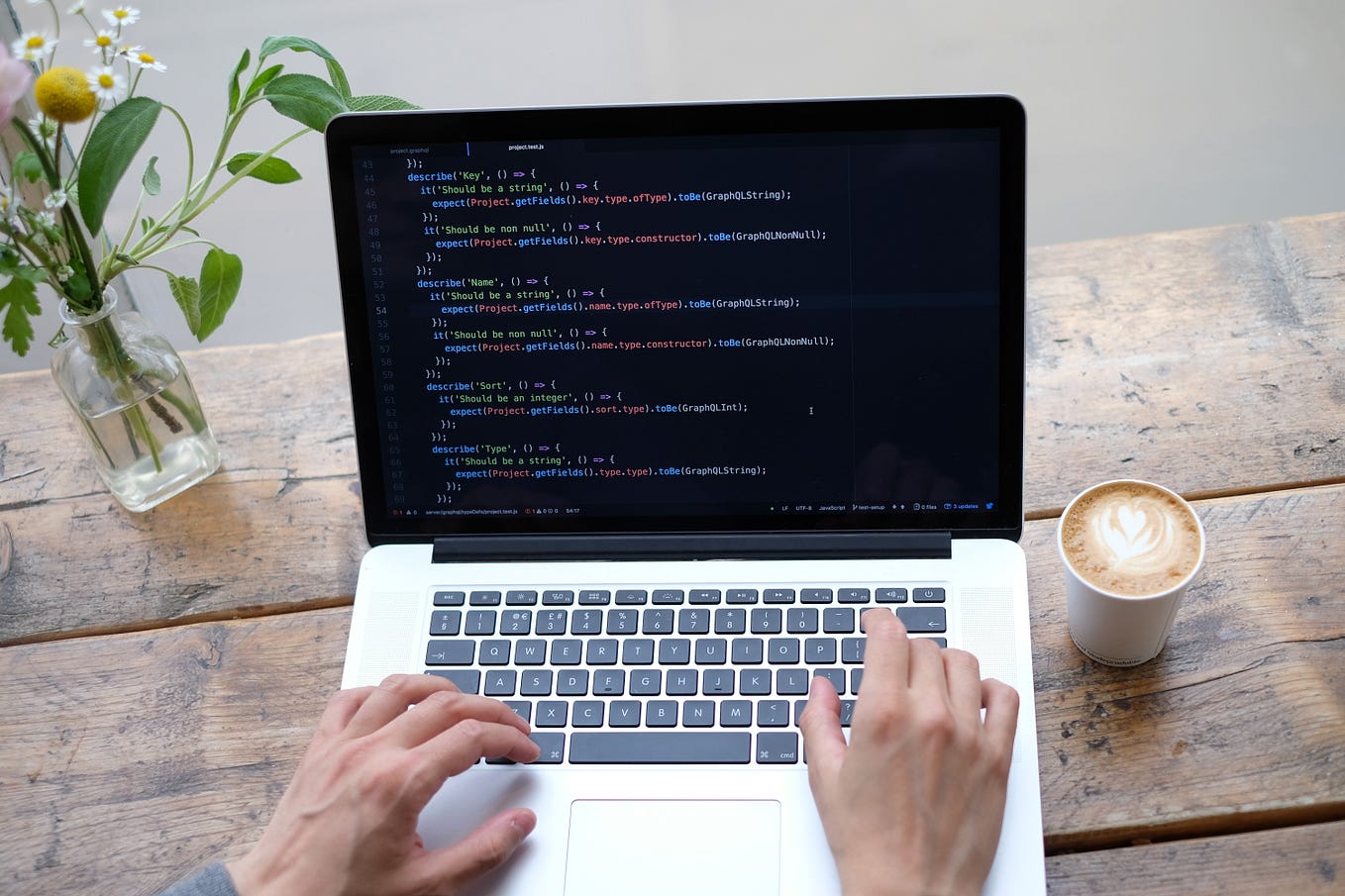
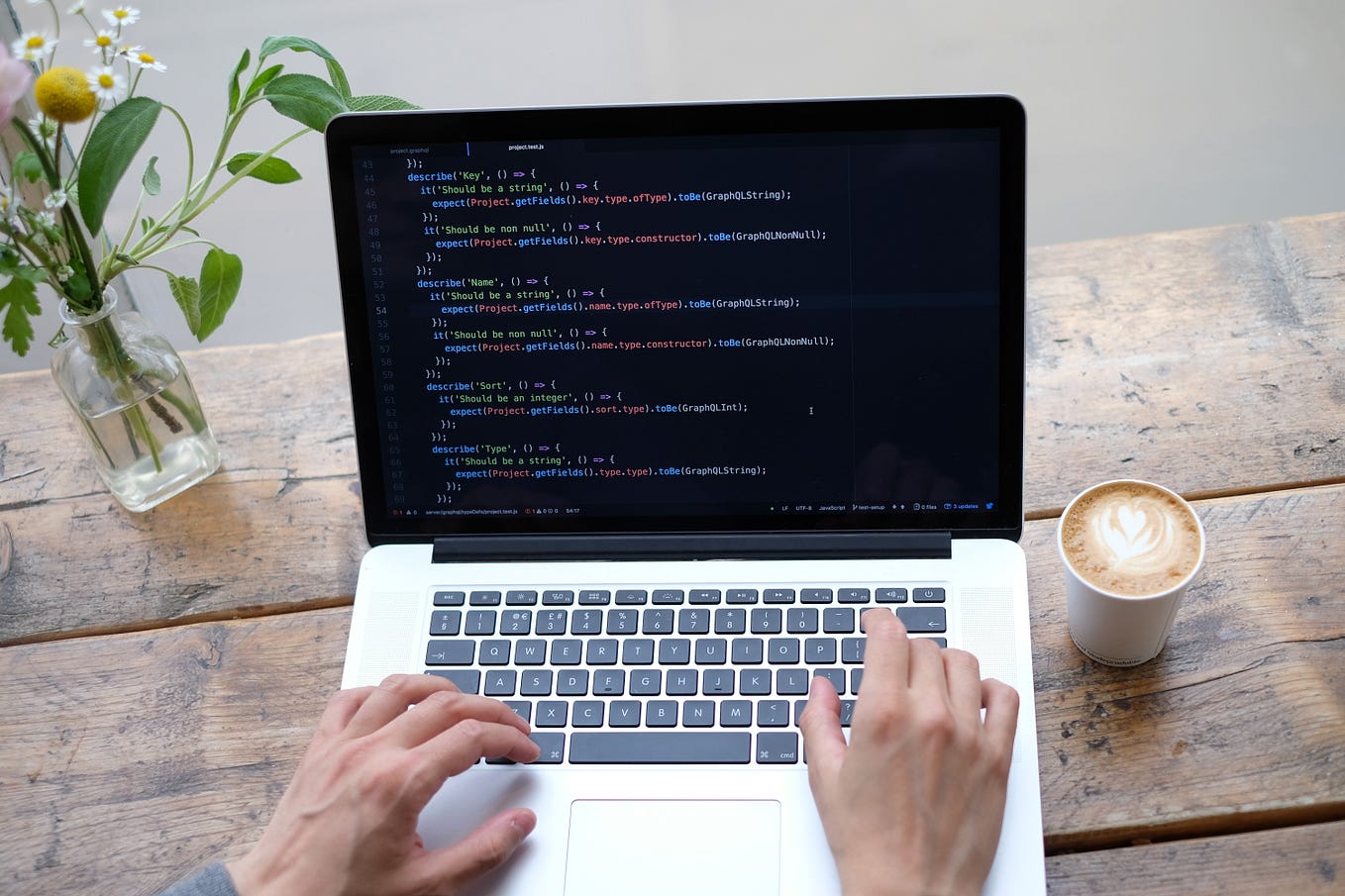
Articles
How To Store Data In Mongodb Using Node.Js
Modified: December 7, 2023
Learn how to store data in MongoDB using Node.js with this comprehensive guide. Explore different strategies and best practices for efficient data storage.
(Many of the links in this article redirect to a specific reviewed product. Your purchase of these products through affiliate links helps to generate commission for Storables.com, at no extra cost. Learn more)
Introduction
In today’s digital age, data is fundamental to the functioning of businesses and organizations. Storing and managing this data efficiently is crucial for the success of any project. MongoDB, with its flexible and scalable document-based data model, has emerged as one of the most popular database systems. When combined with Node.js, a powerful and versatile JavaScript runtime environment, developers can create robust and efficient applications.
This article will guide you through the process of storing data in MongoDB using Node.js. Whether you are a beginner or an experienced developer, this tutorial will provide a step-by-step approach to help you understand the fundamentals of MongoDB and its integration with Node.js.
Before we dive into the implementation details, it is important to have MongoDB and Node.js installed on your system. If you haven’t already done so, let’s start by installing these technologies.
Key Takeaways:
- Seamless Integration
Storing data in MongoDB using Node.js offers a seamless integration, allowing developers to create robust applications with flexible and scalable data management capabilities, ensuring efficient project success. - Comprehensive Data Management
From establishing connections to querying, updating, and deleting data, this article provides a comprehensive guide to effectively manage data in MongoDB using Node.js, empowering developers with essential skills for data manipulation.
Read more: How To Store Data In Database
Installing MongoDB and Node.js
Before we can begin working with MongoDB and Node.js, we need to ensure that they are properly installed on our system.
Installing MongoDB
First, let’s install MongoDB. MongoDB provides comprehensive installation guides for various operating systems on their official website, so be sure to refer to the appropriate guide for your system.
- Go to the MongoDB website and download the appropriate MongoDB Community Edition package for your OS.
- Follow the installation instructions provided in the documentation for your operating system.
- Once MongoDB is successfully installed, make sure to add the MongoDB executable to your system’s PATH variable so that you can access it from any location in the command line.
Installing Node.js
Next, let’s install Node.js, which will allow us to develop applications using JavaScript on the server side.
- Go to the official Node.js website and download the LTS (Long-Term Support) version of Node.js for your operating system.
- Run the installer and follow the installation instructions.
- After successful installation, you can verify the installation by opening a terminal or command prompt and running the command
node -v
. This should display the version number of Node.js installed on your system.
With both MongoDB and Node.js successfully installed on your system, we are now ready to start working with them. In the next section, we will establish a connection to MongoDB from our Node.js application.
Creating a MongoDB Connection
Before we can perform any database operations using Node.js and MongoDB, we need to establish a connection to the MongoDB server. To do this, we will utilize the mongodb
package, which is the official MongoDB driver for Node.js.
To begin, let’s create a new Node.js project by creating a directory and initializing it with npm. Open your terminal or command prompt and follow these steps:
- Create a new directory for your project using the command
mkdir my-project
. - Change into the project directory using
cd my-project
. - Initialize the project with npm using
npm init -y
. The-y
flag will automatically generate apackage.json
file with default values.
Once the project is set up, we can install the necessary dependencies, including the mongodb
package. Run the following command in your terminal:
npm install mongodb
This will install the mongodb
package and add it as a dependency in your package.json
file.
Now that we have the required packages installed, let’s create a connection to MongoDB in our Node.js application. Create a new JavaScript file, such as app.js
, in the root directory of your project and open it in your preferred code editor.
Start by requiring the mongodb
package at the top of the file:
const { MongoClient } = require('mongodb');
Next, define a constant named url
that specifies the connection string for your MongoDB server. This string typically follows the format mongodb://localhost:27017
, where localhost
represents the hostname or IP address of your MongoDB server, and 27017
is the default port for MongoDB.
Now, let’s establish a connection to the server using the MongoClient
class. Add the following code:
const client = new MongoClient(url, { useNewUrlParser: true, useUnifiedTopology: true });
client.connect((err) => {
if (err) {
console.error('Error connecting to MongoDB:', err);
return;
}
console.log('Connected to MongoDB!');
// Perform database operations here
client.close();
});
The connect
method of the MongoClient
class establishes a connection to the MongoDB server specified by the url
. If the connection is successful, the provided callback function will be executed and a success message will be logged in the console.
We have now successfully created a connection to MongoDB in our Node.js application. In the next section, we will learn how to create a database and collection.
Read more: How To Store Data In Python
Creating a Database and Collection
Now that we have established a connection to MongoDB using Node.js, we can proceed with creating a database and collection to store our data. In MongoDB, a database is a container for collections, and a collection is a group of MongoDB documents.
To create a database and collection, we will utilize the db
object returned by the connect
method. Within the callback function of the connect
method, add the following code:
const db = client.db('mydatabase');
const collection = db.collection('mycollection');
The db
object represents the database, and the collection
object represents the collection within that database. Replace mydatabase
with the desired name for your database and mycollection
with the name for your collection.
If the specified database does not exist, MongoDB will automatically create it when documents are inserted.
Now that we have created a database and collection, we can move on to inserting data into MongoDB. In the next section, we will explore how to insert data using Node.js.
Inserting Data into MongoDB
Now that we have established a connection to MongoDB and created a database and collection, we can proceed with inserting data into our collection. In MongoDB, data is represented as documents, which are stored in collections.
To insert a document into MongoDB using Node.js, we will utilize the insertOne
or insertMany
method of the collection object. The insertOne
method is used to insert a single document, while the insertMany
method is used to insert multiple documents at once.
Let’s start by inserting a single document. Within the connect callback function, add the following code:
javascript const document = { name: “John Doe”, email: “johndoe@example.com” }; collection.insertOne(document, (err, result) => { if (err) { console.error(‘Error inserting document:’, err); return; } console.log(‘Document inserted:’, result.insertedId); });
In this example, we define a JavaScript object called `document` representing the data we want to insert. The `insertOne` method is then called on the `collection` object, with the `document` as the first argument. A callback function is provided to handle the result of the insertion.
If the insertion is successful, the result object will contain information about the inserted document, including its `_id` (unique identifier). We log the generated `_id` in the console after successful insertion.
If you want to insert multiple documents at once, you can use the `insertMany` method. The syntax is similar, but you pass an array of documents as the first argument:
javascript const documents = [ { name: “Jane Smith”, email: “janesmith@example.com” }, { name: “Robert Johnson”, email: “robertjohnson@example.com” }, // more documents… ]; collection.insertMany(documents, (err, result) => { if (err) { console.error(‘Error inserting documents:’, err); return; } console.log(‘Documents inserted:’, result.insertedCount); });
Here, we define an array of documents and pass it to the `insertMany` method. The callback function will provide information about the inserted documents, including the `insertedCount` property indicating the number of documents successfully inserted.
We have now learned how to insert data into MongoDB using Node.js. In the next section, we will explore how to query data from MongoDB.
Querying Data from MongoDB
Once we have inserted data into MongoDB, it’s important to be able to retrieve that data efficiently. In MongoDB, data retrieval is performed using queries, which allow us to filter and retrieve specific documents based on certain criteria.
To query data from MongoDB using Node.js, we will utilize the various query methods provided by the collection object, such as the `find` method.
Let’s start by querying for all documents in a collection. Within the connect callback function, add the following code:
javascript collection.find().toArray((err, documents) => { if (err) { console.error(‘Error querying documents:’, err); return; } console.log(‘Retrieved documents:’, documents); });
In this example, the `find` method is called without any arguments, which retrieves all documents in the collection. The `toArray` method is chained to convert the retrieved cursor into an array of documents.
If the query is successful, the retrieved documents will be logged in the console.
If you want to retrieve documents that match specific criteria, you can pass a query object to the `find` method. For example, to retrieve documents where the name is “John Doe”, you can modify the query as follows:
javascript const query = { name: “John Doe” }; collection.find(query).toArray((err, documents) => { if (err) { console.error(‘Error querying documents:’, err); return; } console.log(‘Retrieved documents:’, documents); });
In this case, we provide a query object that specifies the criteria for the document retrieval. Only documents that match the specified criteria will be returned.
Additionally, you can use various query operators, such as `$gt`, `$lt`, and `$regex`, to perform more complex queries and retrieve specific documents based on your needs.
We have now learned how to query data from MongoDB using Node.js. In the next section, we will explore how to update data in MongoDB.
When storing data in MongoDB using Node.js, make sure to properly structure your data to take advantage of MongoDB’s document-oriented nature. Use Mongoose to define schemas and models for your data to ensure consistency and validation.
Updating Data in MongoDB
Updating data in MongoDB is a common operation when working with databases. With Node.js and MongoDB, we can easily update existing documents in collections using the update methods provided by the collection object.
Let’s explore how to update data in MongoDB using Node.js. Within the connect callback function, add the following code:
javascript const filter = { name: “John Doe” }; const update = { $set: { email: “johndoe@example.com” } }; collection.updateOne(filter, update, (err, result) => { if (err) { console.error(‘Error updating document:’, err); return; } console.log(‘Document updated:’, result.modifiedCount); });
In this example, we define a filter object that specifies the criteria for the document we want to update. In this case, we want to update the document with the name “John Doe”. The update object specifies the changes to be made using the `$set` operator. In this example, we update the email field of the document.
The `updateOne` method is called with the filter and update objects as arguments, along with a callback function to handle the result of the update operation.
If the update is successful, the result object will contain information about the modified document, including the `modifiedCount` property indicating the number of documents updated. We log this information in the console.
Similar to updating a single document, we can also update multiple documents using the `updateMany` method. The syntax is similar, but you need to provide a filter object and an update object:
javascript const filter = { category: “Books” }; const update = { $set: { price: 19.99 } }; collection.updateMany(filter, update, (err, result) => { if (err) { console.error(‘Error updating documents:’, err); return; } console.log(‘Documents updated:’, result.modifiedCount); });
In this example, we update all documents with the category “Books” by setting their price to 19.99.
We have now learned how to update data in MongoDB using Node.js. In the next section, we will explore how to delete data from MongoDB.
Read more: How To Store Research Data Securely
Deleting Data from MongoDB
Deleting data from MongoDB is a crucial operation when it comes to managing databases. With Node.js and MongoDB, we can easily remove documents from collections using the delete methods provided by the collection object.
Let’s explore how to delete data from MongoDB using Node.js. Within the connect callback function, add the following code:
javascript const filter = { name: “John Doe” }; collection.deleteOne(filter, (err, result) => { if (err) { console.error(‘Error deleting document:’, err); return; } console.log(‘Document deleted:’, result.deletedCount); });
In this example, we define a filter object that specifies the criteria for the document we want to delete. In this case, we want to delete the document with the name “John Doe”.
The `deleteOne` method is called with the filter object as an argument, along with a callback function to handle the result of the deletion operation.
If the deletion is successful, the result object will contain information about the deleted document, including the `deletedCount` property indicating the number of documents deleted. We log this information in the console.
In addition to deleting a single document, we can also delete multiple documents using the `deleteMany` method. The syntax is similar, but you need to provide a filter object:
javascript const filter = { category: “Books” }; collection.deleteMany(filter, (err, result) => { if (err) { console.error(‘Error deleting documents:’, err); return; } console.log(‘Documents deleted:’, result.deletedCount); });
In this example, we delete all documents with the category “Books”.
We have now learned how to delete data from MongoDB using Node.js. In the next section, we will conclude our discussion and summarize the key points we covered.
Conclusion
In this article, we have explored the process of storing data in MongoDB using Node.js. We started by installing MongoDB and Node.js, ensuring that both were properly set up on our system. We then proceeded to create a MongoDB connection in our Node.js application, establishing a connection to the MongoDB server.
Once the connection was established, we learned how to create a database and collection, providing a container for our data. We then delved into the process of inserting data into MongoDB, using both the insertOne
and insertMany
methods to add documents to our collection.
Next, we covered the topic of querying data from MongoDB. We explored using the find
method to retrieve documents, both all documents and those that matched specific criteria based on our queries.
After querying data, we moved on to updating existing data in MongoDB. We learned how to use the updateOne
and updateMany
methods to modify the fields of documents, ensuring that our data remains up to date and accurate.
Lastly, we discussed the process of deleting data from MongoDB using the deleteOne
and deleteMany
methods. We explored how to remove documents from our collection based on specified criteria.
By following the concepts and techniques covered in this article, you are now armed with the knowledge to effectively store, retrieve, update, and delete data in MongoDB using Node.js.
Remember to practice and experiment with these concepts, as hands-on experience will enhance your understanding and proficiency in using MongoDB and Node.js for data management.
Happy coding!
Frequently Asked Questions about How To Store Data In Mongodb Using Node.Js
Was this page helpful?
At Storables.com, we guarantee accurate and reliable information. Our content, validated by Expert Board Contributors, is crafted following stringent Editorial Policies. We're committed to providing you with well-researched, expert-backed insights for all your informational needs.
0 thoughts on “How To Store Data In Mongodb Using Node.Js”