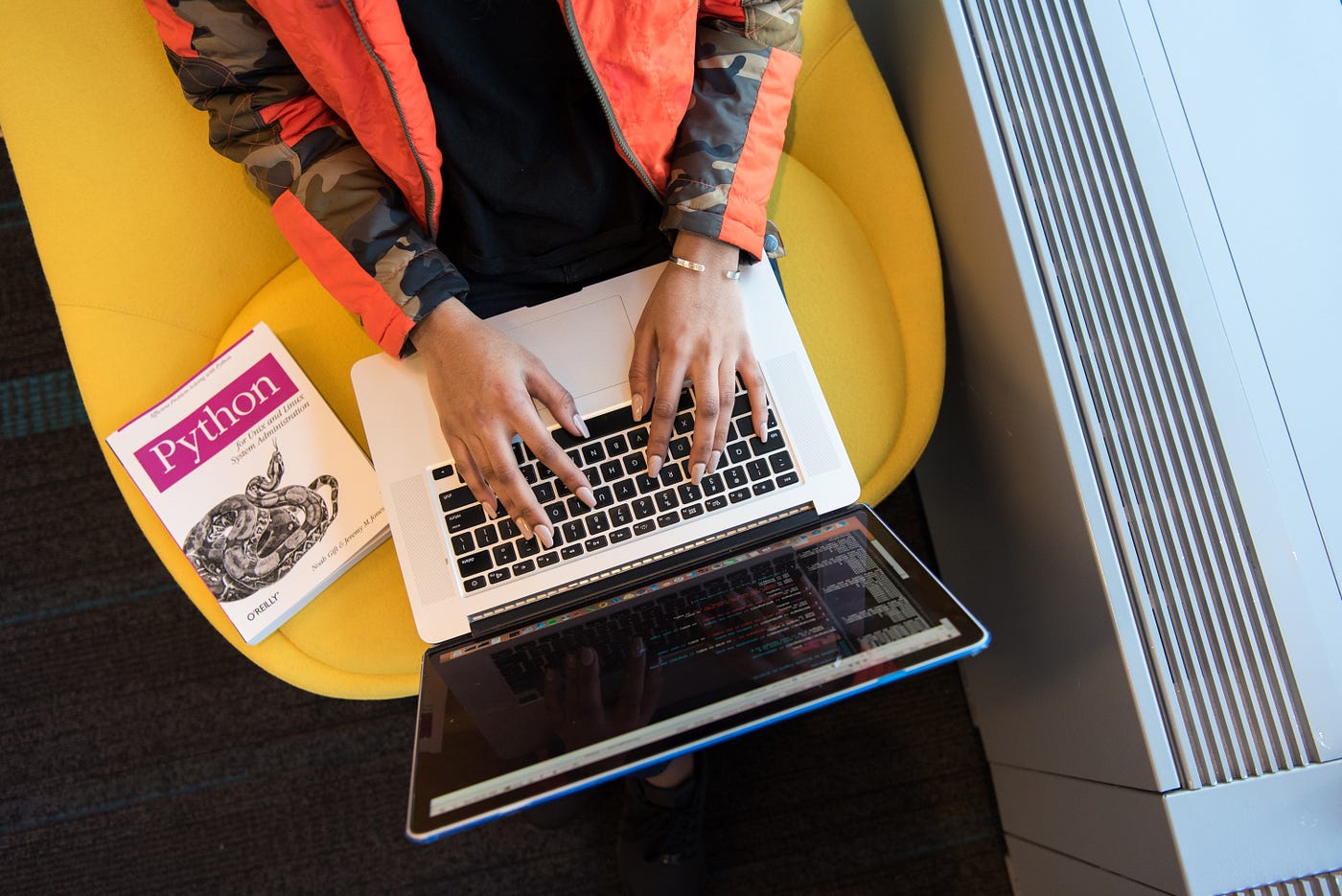
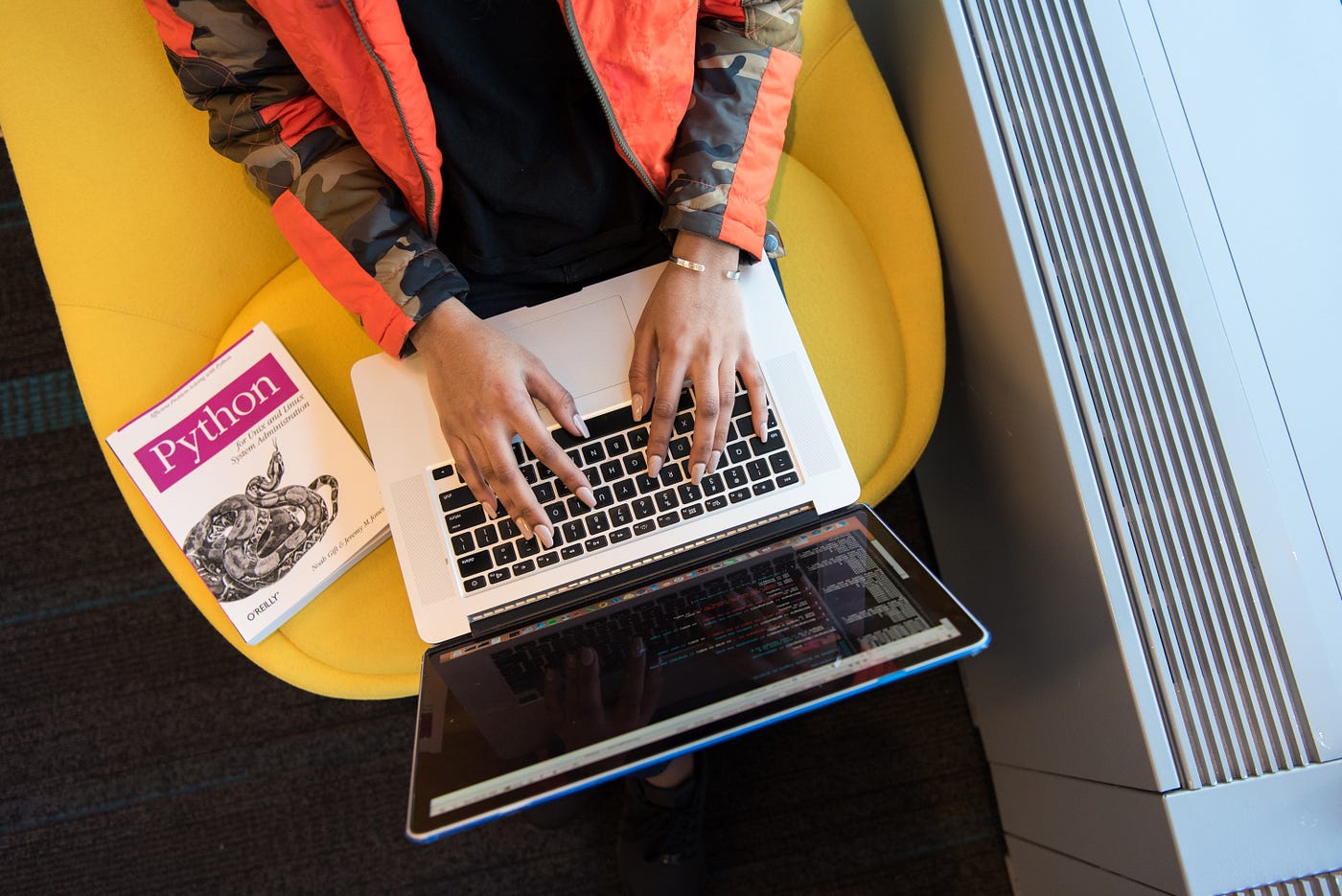
Articles
How To Store Data In Python
Modified: December 7, 2023
Learn how to store and manage data efficiently in Python with our informative articles. Find step-by-step guides and tutorials on data storage methods and techniques.
(Many of the links in this article redirect to a specific reviewed product. Your purchase of these products through affiliate links helps to generate commission for Storables.com, at no extra cost. Learn more)
Introduction
When working with Python, one of the fundamental tasks is storing data. Whether you’re working on a small personal project or a large-scale application, the ability to store and manage data efficiently is crucial. Python offers various methods and data structures that allow you to store and organize data in a way that meets your specific needs.
In this article, we will explore different techniques for storing data in Python. We will start by looking at how to store data in variables, followed by an exploration of more complex data structures such as lists, dictionaries, sets, and tuples. Additionally, we’ll discuss how to store data in files and take a brief look at working with databases for data storage.
By the end of this article, you will have a solid understanding of various methods for storing data in Python and be able to choose the most suitable approach depending on your specific use case.
Key Takeaways:
- Python offers versatile data storage options, from simple variables to complex structures like lists, dictionaries, sets, and tuples. Choose the right method based on your data’s size, operations, and security needs.
- Whether storing data in files for persistence or using databases for scalability, Python provides efficient solutions. Consider factors like access time, memory usage, and data integrity to optimize your data storage decisions.
Read more: How To Store Data In Database
Storing Data in Python Variables
In Python, variables are the simplest way to store data. A variable is like a container that holds a value, which can be of any data type such as numbers, strings, or even more complex objects.
To store data in a variable, you can use the assignment operator (=). For example:
name = “John”
age = 25
In the above example, we assigned the value “John” to the variable `name` and the value 25 to the variable `age`.
Variables are particularly useful when you need to store and manipulate a single piece of data. However, they have limited capabilities when it comes to organizing and managing larger datasets.
Next, let’s explore more powerful data structures that can handle multiple values and provide more flexibility for data storage.
Using Lists to Store Data
Lists are one of the most common and versatile data structures in Python. They are used to store an ordered collection of items, where each item can be of any data type.
To create a list, you can enclose the items in square brackets and separate them with commas. For example:
fruits = [“apple”, “banana”, “orange”]
In the above example, we created a list called `fruits` with three elements: “apple”, “banana”, and “orange”.
Lists are mutable, which means you can modify their elements by accessing them using their index position. For example, to change the first element of the `fruits` list to “grape”, you can do the following:
fruits[0] = “grape”
Lists also provide various built-in methods to manipulate and access their elements. Some common operations include:
- Appending an element to the end of the list using the `append()` method
- Inserting an element at a specific index position using the `insert()` method
- Removing an element by its value using the `remove()` method
- Sorting the elements in the list using the `sort()` method
- Accessing a subset of elements using slicing
Lists offer flexibility and efficiency when it comes to storing and manipulating collections of data. However, they may not be the most suitable choice if you need to associate values with specific keys. In such cases, dictionaries can provide a more suitable solution.
Storing Data in Dictionaries
Dictionaries are another powerful data structure in Python that allow you to store values in key-value pairs. Unlike lists, which use integer indices to access elements, dictionaries use keys that can be of any immutable data type, such as strings or numbers.
To create a dictionary, you enclose key-value pairs in curly braces and separate them with commas. Each key-value pair is written as `key: value`. For example:
student = {
“name”: “John”,
“age”: 25,
“major”: “Computer Science”
}
In the above example, we created a dictionary called `student` with three key-value pairs representing a student’s name, age, and major.
Accessing values in a dictionary is done by specifying the key in square brackets. For example, to access the value of the “name” key in the `student` dictionary, you can use:
name = student[“name”]
Dictionaries provide a flexible and efficient way to organize and access data based on meaningful keys. They also offer built-in methods to modify, add, or remove key-value pairs. Some commonly used methods include:
- Adding a new key-value pair using the `update()` method or direct assignment
- Modifying the value of an existing key using direct assignment
- Removing a key-value pair using the `pop()` method or the `del` keyword
With dictionaries, you can easily associate multiple values with a single entity, making them ideal for scenarios where you need to store data with meaningful labels or attributes.
Working with Sets for Data Storage
In addition to lists and dictionaries, Python provides a built-in data structure called a set. A set is an unordered collection of unique elements that can be of any hashable data type.
To create a set, you can enclose elements in curly braces or use the `set()` constructor. For example:
fruits = {“apple”, “banana”, “orange”}
In the above example, we created a set called `fruits` with three unique elements: “apple”, “banana”, and “orange”.
One of the key features of sets is that they automatically remove duplicate elements. If you try to add a duplicate element to a set, it will be ignored. This makes sets useful for tasks such as finding unique values or removing duplicates from a collection.
Sets also support various set operations, such as union, intersection, and difference. These operations allow you to combine sets or find common or distinct elements between sets.
Some common set operations include:
- Adding elements to a set using the `add()` method
- Removing elements from a set using the `remove()` method or the `discard()` method
- Finding the union of two or more sets using the `union()` method or the `|` operator
- Finding the intersection of two or more sets using the `intersection()` method or the `&` operator
- Finding the difference between two sets using the `difference()` method or the `-` operator
Sets provide a convenient and efficient way to perform operations on unique elements. However, they do not preserve the order of elements and do not support indexing, which may limit their applicability in certain scenarios.
Use Python’s built-in data structures like lists, dictionaries, and sets to store and organize your data. These structures offer efficient ways to access, modify, and manipulate your data.
Read more: How To Store Research Data Securely
Using Tuples as Data Containers
In Python, tuples are another data structure that can be used to store related pieces of data. Tuples are similar to lists but are immutable, meaning their elements cannot be modified after they are created.
To create a tuple, you can enclose elements in parentheses and separate them with commas. For example:
person = (“John”, 25, “New York”)
In the above example, we created a tuple called `person` with three elements representing a person’s name, age, and hometown.
Tuples provide a way to group related data together and ensure its immutability, which can be beneficial in scenarios where you want to prevent accidental modification of the data.
Accessing elements in a tuple is similar to accessing elements in a list, using indexing. For example, to access the first element in the `person` tuple, you can use:
name = person[0]
While tuples cannot be modified, you can create new tuples by concatenating or slicing existing tuples.
Tuples are often used in situations where you want to represent a fixed collection of related data. They can also be helpful when you need to pass multiple values to a function or when you want to ensure the integrity of data that should not be changed.
Storing Data in Files
In addition to in-memory data structures, Python also provides the ability to store data in files. This allows you to persist your data even after the program terminates and provides a way to share data between different programs or systems.
There are several ways to store data in files in Python. The most common methods include:
- Plain Text Files: Plain text files store data in a human-readable format. You can use functions like `open()` to open a file and `read()` or `write()` to read from or write to the file. Plain text files are suitable for storing simple data such as strings or numerical values.
- CSV Files: CSV (Comma-Separated Values) files are commonly used for storing tabular data. Python provides the `csv` module, which simplifies reading from or writing to CSV files. CSV files are useful when working with spreadsheets or when you need to exchange data with other applications or systems.
- JSON Files: JSON (JavaScript Object Notation) files are used to store structured data in a human-readable format. Python has a built-in `json` module that makes it easy to read from or write to JSON files. JSON files are widely used for data exchange between different programming languages and systems.
- Binary Files: Binary files store data in a compact binary format and are typically used for storing complex data structures or large amounts of data. Python provides functions like `pickle` to serialize and deserialize data structures into binary files. Binary files are suitable when you need to preserve the exact state of the data and its structure.
When working with files, it’s important to handle exceptions and close the file properly to ensure the integrity of the data. It’s also a good practice to consider security aspects, such as file permissions and data encryption, depending on the sensitivity of the data stored.
Working with Databases for Data Storage
When dealing with larger datasets or more complex data structures, using a database for data storage is often a more efficient and scalable solution. Python provides various libraries and modules that allow you to interact with different database systems.
Here are some common steps involved in working with databases:
- Connecting to the Database: First, you need to establish a connection to the database by providing the necessary credentials and connection parameters. Python offers libraries such as
sqlite3
,MySQLdb
, andpsycopg2
to connect to SQLite, MySQL, and PostgreSQL databases, respectively. - Creating a Table: Once connected, you can create a table to define the structure of your data. This includes specifying the column names, data types, and any constraints. You can use SQL statements or libraries like
sqlalchemy
to work with databases in a more object-oriented manner. - Inserting Data: After creating the table, you can insert data into it using SQL
INSERT
statements or ORM (Object-Relational Mapping) methods provided by libraries likesqlalchemy
. - Querying Data: Once data is inserted, you can retrieve it from the database using SQL
SELECT
statements or ORM methods. You can filter, sort, and aggregate the data based on your requirements. - Updating and Deleting Data: If you need to modify or remove data from the database, you can use SQL
UPDATE
andDELETE
statements or corresponding ORM methods.
Using databases not only provides efficient data storage but also offers advanced features like indexing, data consistency, and concurrency control. It enables you to manage large amounts of data, perform complex queries, and ensure data integrity.
It’s worth noting that each database system has its own syntax and features. Therefore, it’s essential to refer to the specific documentation of the database and Python library you are working with.
Conclusion
Storing data is a critical aspect of any programming task, and Python offers a range of techniques and data structures to suit different needs. From simple variables to more complex data structures like lists, dictionaries, sets, and tuples, Python provides flexibility and efficiency in managing data.
When dealing with larger datasets or the need for data persistence, storing data in files or using databases becomes essential. File handling allows you to store and share data in plain text, CSV, JSON, or binary formats, while databases offer advanced features for efficient data management, storage, retrieval, and manipulation.
By understanding these various methods, you can choose the right approach for your specific needs. Consider the size and structure of your data, the type of operations you’ll perform, and the scalability and security requirements of your project.
Remember to optimize your data storage decisions by considering factors such as access time, memory usage, and data integrity. Additionally, ensure to follow best practices like closing file connections, handling exceptions, and securing sensitive data when working with files and databases.
With a solid grasp of data storage techniques in Python, you are now equipped to handle a wide range of data management tasks efficiently and effectively.
Frequently Asked Questions about How To Store Data In Python
Was this page helpful?
At Storables.com, we guarantee accurate and reliable information. Our content, validated by Expert Board Contributors, is crafted following stringent Editorial Policies. We're committed to providing you with well-researched, expert-backed insights for all your informational needs.
0 thoughts on “How To Store Data In Python”