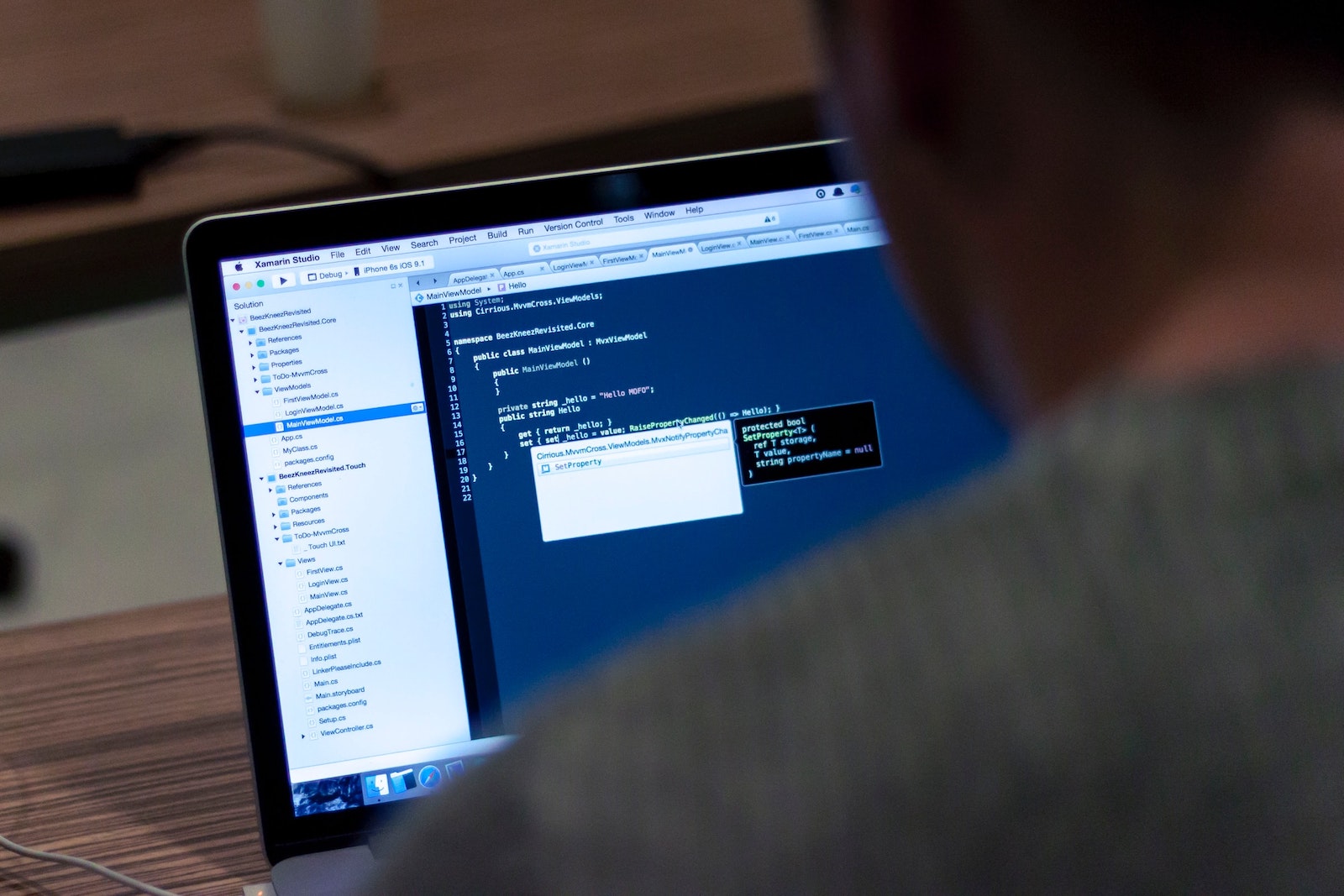
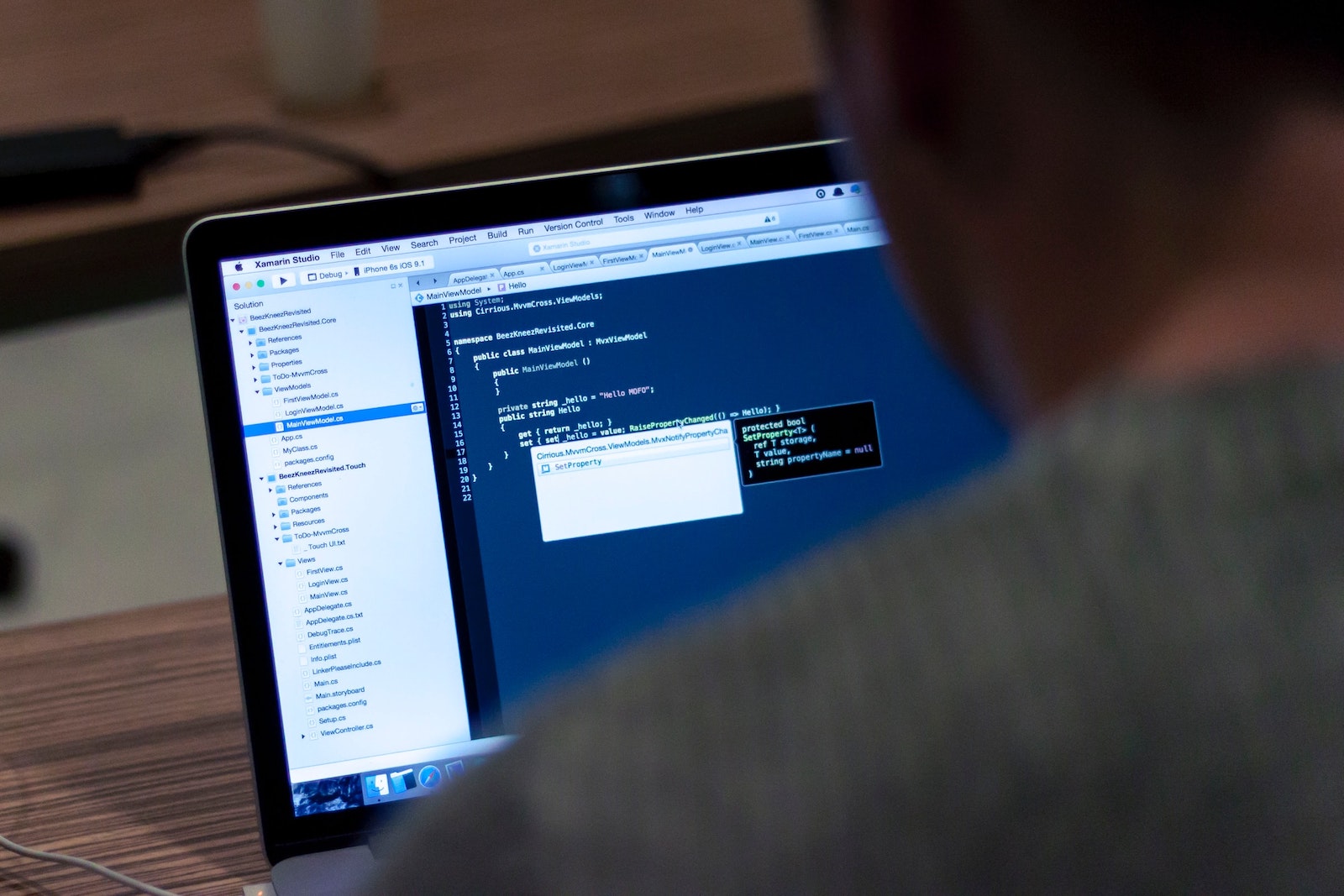
Articles
How To Store Data In Java
Modified: December 7, 2023
Learn how to store and manipulate data effectively in Java with our informative articles. Explore various techniques and best practices for efficient data storage.
(Many of the links in this article redirect to a specific reviewed product. Your purchase of these products through affiliate links helps to generate commission for Storables.com, at no extra cost. Learn more)
Introduction
When working with Java, storing data is an essential part of any application development process. Whether it is simple variables, complex objects, or large datasets, efficient and reliable data storage is crucial for the smooth functioning of your application.
In this article, we will explore different ways to store data in Java. We will examine both the built-in options provided by the language itself, such as arrays and collections, as well as external options like databases, serialization, and JSON/XML.
Understanding data storage in Java is essential for developers to make informed decisions about the best approach for their projects. By learning various storage options, you can optimize your application’s performance, ensure data integrity, and easily retrieve and manipulate data as needed.
Throughout this article, we will dive deep into each storage method, exploring their characteristics, use cases, advantages, and considerations. By the end, you will have a comprehensive understanding of the different ways to store data in Java, allowing you to choose the most appropriate solution for your specific application requirements. So, let’s get started!
Key Takeaways:
- Choose the Right Storage Method
Understanding the strengths and limitations of different data storage options in Java is crucial for efficient application development. Consider factors like data volume, performance requirements, and persistence needs to make informed decisions. - Combine Storage Methods for Optimization
Optimize data storage in Java applications by combining built-in options like arrays and collections with external options such as databases, serialization, and JSON/XML formats. Each method has its strengths, and leveraging them together can create robust and effective data storage solutions.
Read more: How To Store Data In Database
Understanding Data Storage in Java
Before we delve into the various data storage options in Java, it is crucial to understand the concept of data storage itself. Data storage refers to the mechanisms and techniques used to store and organize data for efficient access and retrieval.
In Java, data can be stored in different data structures, ranging from simple variables to complex objects and databases. Each data storage option has its own characteristics and use cases, and understanding them will help you make informed decisions when designing and implementing your applications.
One fundamental aspect of data storage is the ability to store and retrieve data quickly and efficiently. This is achieved by utilizing data structures that provide fast access and manipulation operations. Different data structures in Java offer different performance characteristics, such as arrays providing constant-time access for indexed data and collections providing dynamic resizing and flexible operations on elements.
Another important consideration is the persistence of data. In some cases, you may need the data to be stored permanently, even after the application exits or the system restarts. This is where external data storage options like files and databases come into play. These options allow data to be stored persistently and accessed later, ensuring data integrity and availability.
Data storage in Java also involves considerations such as data organization, security, and scalability. Depending on the nature of your application and the volume of data you need to store, you may need to choose storage options that provide efficient organization and retrieval mechanisms. Additionally, data security is essential to protect sensitive information and prevent unauthorized access. Scalability refers to the ability to handle large amounts of data efficiently as your application grows.
By understanding these aspects of data storage in Java, you can make informed decisions when selecting the most appropriate storage option for your application. In the following sections, we will explore the different options available and discuss their characteristics, use cases, and best practices.
Built-in Data Storage Options
Java provides several built-in data storage options that are part of the standard library. These options include arrays and collections, which are widely used for storing and manipulating data in Java applications.
Arrays: Arrays in Java are fixed-size, contiguous data structures that store elements of the same type. They offer efficient direct access to elements based on their index. Arrays are ideal for storing a fixed number of elements of known types. However, they require contiguous memory allocation, so their size cannot be dynamically changed once created.
Collections: The Java Collections Framework provides a set of classes and interfaces for storing and manipulating collections of objects. Collections offer more flexibility than arrays, as they can dynamically resize and provide operations like adding, removing, and searching elements. Some commonly used collection classes include ArrayList, LinkedList, and HashSet, each with its own characteristics and use cases.
These built-in data storage options are suitable for small to medium-sized datasets where the data structure can be predefined or dynamically altered. They provide efficient operations for managing data in memory and are commonly used in algorithm design, data processing, and other general programming scenarios.
When choosing between arrays and collections, consider the specific requirements of your application. If you need a fixed-size data structure with direct access to elements, arrays are a suitable choice. On the other hand, if you require dynamic resizing, additional functionality, and ease of use, collections are the way to go.
While arrays and collections are powerful built-in options, they have limitations. They are primarily meant for in-memory storage and are not suitable for long-term persistence of data. If you need to store data permanently or handle larger datasets, you will need to explore external data storage options like files or databases, which we will discuss in the next section.
Arrays
Arrays are one of the basic built-in data storage options in Java. They provide a fixed-size, contiguous block of memory to store elements of the same type. Each element in the array can be accessed using an index, which starts from 0 for the first element.
Arrays are ideal for scenarios where you have a fixed number of elements of the same type and want efficient direct access to them. They are widely used in various algorithms and data structures due to their simplicity and performance advantages.
To create an array in Java, you need to specify the type of elements it will store and the desired size. For example, to create an array of integers with a size of 5:
java
int[] numbers = new int[5];
You can also initialize an array with predefined values:
java
int[] numbers = { 1, 2, 3, 4, 5 };
Once created, you can access individual elements using the index. For example, to access the second element of the array:
java
int secondNumber = numbers[1];
Arrays provide constant-time access to elements, as accessing an element by its index does not depend on the size of the array. However, they have some limitations. One limitation is that the size of an array is fixed upon creation and cannot be changed dynamically. If you need a dynamic data structure that can grow or shrink in size, consider using collections instead.
Another limitation is that arrays are primarily meant for in-memory storage. They do not offer built-in mechanisms for persisting data to disk or retrieving data from external sources. If you need to store arrays permanently or handle larger datasets, you will need to explore other data storage options such as files or databases.
Despite these limitations, arrays are still widely used in Java programming. They provide a simple and efficient way to store and manipulate data when the size is fixed and direct access is required.
Collections
Collections are a powerful built-in data storage option in Java that provide dynamic-sized data structures for storing and manipulating objects. The Java Collections Framework includes a wide range of classes and interfaces that offer different functionalities and characteristics.
Some commonly used collection classes include:
- ArrayList: An implementation of the List interface that uses an underlying array to store elements. ArrayList provides dynamic resizing, allowing elements to be added or removed without worrying about the size limitations of arrays.
- LinkedList: A doubly-linked list implementation of the List interface. LinkedList provides efficient insertion and deletion operations in the middle of the list, but slower random access compared to ArrayList.
- HashSet: An implementation of the Set interface that stores elements using a hash table. HashSet does not allow duplicate elements and provides constant-time add, remove, and contains operations.
- TreeSet: An implementation of the Set interface that stores elements in a sorted tree structure. TreeSet maintains elements in sorted order, allowing efficient operations like finding the smallest or largest element.
These are just a few examples of the many collection classes available in the Java Collections Framework. Each class provides specific features and performance characteristics, allowing you to choose the most suitable one based on your needs.
Collections offer several advantages over arrays. Firstly, they can dynamically resize, allowing you to add or remove elements as needed without worrying about the fixed size limitation of arrays. This flexibility makes collections more convenient for scenarios where the number of elements may vary or is unknown in advance.
Secondly, collections provide various operations and algorithms to manipulate the stored elements efficiently. They offer methods for adding, removing, searching, sorting, and iterating over elements, making it easier to work with complex data structures.
Additionally, collections can store objects of different types and utilize Java’s object-oriented features. This flexibility allows you to build more complex data structures that can hold and manage heterogeneous data.
However, collections also have their downsides. They consume more memory compared to arrays due to the overhead of extra object references and internal structures. They are also slightly slower than arrays for direct access to elements since they involve additional method calls and dynamic dispatch.
Despite these downsides, collections are widely used in Java programming due to their flexibility, extensive functionality, and convenience. They are suitable for a wide range of scenarios that require dynamic data structures and efficient operations on elements.
Read more: How To Store Data In Python
Files
When it comes to storing data persistently and accessing it across multiple sessions of your Java application, working with files is a common and essential approach. Java provides a robust and flexible API for interacting with files and directories, offering various functionalities for reading, writing, and manipulating data.
The java.io.File
class is a fundamental component of Java’s file handling capabilities. It represents a file or directory on the file system, and it provides methods for creating, deleting, renaming, and searching files.
To read data from a file, you can use various I/O classes like FileInputStream
or BufferedReader
. These classes allow you to read data from a file byte by byte or line by line, respectively.
Here is an example of how to read data from a file using a BufferedReader
:
java
try (BufferedReader reader = new BufferedReader(new FileReader(“filename.txt”))) {
String line;
while ((line = reader.readLine()) != null) {
// Process each line of data
}
} catch (IOException e) {
// Handle exception
}
To write data to a file, you can use classes like FileOutputStream
or BufferedWriter
. These classes allow you to write data byte by byte or line by line, respectively.
Here is an example of how to write data to a file using a BufferedWriter
:
java
try (BufferedWriter writer = new BufferedWriter(new FileWriter(“filename.txt”))) {
writer.write(“Hello, world!”);
} catch (IOException e) {
// Handle exception
}
In addition to basic file handling, Java also provides advanced functionality for manipulating directories, copying or moving files, and performing file system operations. The java.nio.file
package offers a more modern and powerful API for working with files and directories.
When working with files, it is crucial to handle exceptions properly, as file operations can throw various I/O-related exceptions. It is recommended to use the try-with-resources statement or handle exceptions gracefully to ensure proper resource management and error handling.
Working with files provides the flexibility to store and retrieve data persistently, making it suitable for scenarios where data needs to be preserved between sessions or shared across multiple applications. However, files may not be the optimal solution for handling large or structured datasets. In such cases, databases or other external data storage options may be more appropriate.
Overall, the file handling capabilities of Java empower developers to perform efficient and reliable data storage and retrieval operations, making it a valuable tool in application development.
When storing data in Java, consider using the appropriate data structure such as arrays, lists, maps, or sets based on the specific requirements of your application. Each data structure has its own advantages and can be used to optimize performance and memory usage.
External Data Storage Options
In addition to the built-in data storage options offered by Java, there are external solutions available that provide more advanced and scalable data storage capabilities. These options are particularly useful when working with larger datasets, requiring long-term data persistence, or dealing with structured data.
Let’s explore some of the commonly used external data storage options:
- Databases: Databases are specialized software systems designed for the efficient storage, retrieval, and management of structured data. They offer powerful querying capabilities, transaction support, data integrity enforcement, and scalability. Java supports various database management systems (DBMS) like MySQL, Oracle, PostgreSQL, and more. You can interact with databases using Java Database Connectivity (JDBC) APIs or object-relational mapping (ORM) frameworks like Hibernate.
- Serialization: Serialization is a mechanism to convert objects into a stream of bytes that can be stored in files or sent over the network. Java provides built-in support for object serialization and deserialization through the
java.io.Serializable
interface. Serialization allows you to store complex objects and retrieve them later without losing their state. However, it’s important to note that serialization is not suitable for long-term or cross-language data storage. - JSON/XML: JSON (JavaScript Object Notation) and XML (eXtensible Markup Language) are popular formats for representing structured data. They provide a flexible and human-readable way to store and exchange data. Java includes libraries like Jackson and Gson for working with JSON, and libraries like JAXB for working with XML. These formats are often used for data interchange between different systems or for configuration storage.
Each external data storage option has its own advantages and considerations. Databases offer sophisticated querying capabilities, data integrity enforcement, and scalability. Serialization allows you to store complex objects with their state intact. JSON and XML provide flexible and human-readable data representation. When selecting an external storage option, consider factors such as data volume, performance requirements, data structure, querying needs, and persistence requirements.
It is worth mentioning that external data storage options often require additional libraries, configuration, and infrastructure setup. You may need to install and configure a database server, add libraries for object serialization or JSON/XML processing, and design appropriate data models or schemas.
Choosing the right external data storage option for your Java application depends on the specific requirements of your project. Understanding the strengths and limitations of each option will help you select the most suitable solution to effectively store and retrieve data.
Databases
Databases are a powerful external data storage option widely used in Java applications. They provide a structured and efficient way to store, retrieve, and manage large amounts of data. Databases offer various features and capabilities that make them ideal for handling complex data structures and performing advanced queries.
Java supports different types of databases, including relational databases (such as MySQL, Oracle, PostgreSQL), NoSQL databases (such as MongoDB, Cassandra), and in-memory databases (such as H2, Apache Derby). Relational databases are the most commonly used type, known for their ability to manage structured data using tables, rows, and columns based on a predefined schema.
Java applications can interact with databases using the Java Database Connectivity (JDBC) API, which allows you to establish connections to a database, send queries, and process the results. JDBC provides a standard way to work with various databases, making it possible to write database-agnostic code.
Database management systems (DBMS) offer several advantages:
- Data Integrity: DBMS enforce data integrity through the use of constraints, ensuring that data follows predefined rules. This prevents inconsistent or invalid data from being stored in the database.
- Efficient Retrieval and Querying: Databases provide efficient mechanisms for retrieving and querying data based on specific criteria. SQL (Structured Query Language) is commonly used to write queries that allow you to filter, sort, join, and manipulate data.
- Concurrency Control and Transaction Management: Databases handle concurrent access to data by enforcing locks and managing transactions. This ensures that multiple users can access and modify data without conflicts or inconsistencies.
- Scalability: Databases can handle large volumes of data and support scaling options like replication and sharding to distribute the workload across multiple servers.
When working with databases, it’s important to design an appropriate data model or schema that represents the structure and relationships of your data. This involves defining tables, columns, primary keys, foreign keys, and constraints. Proper database design ensures efficient query execution and data organization.
In addition, Object-Relational Mapping (ORM) frameworks like Hibernate, JPA (Java Persistence API), or MyBatis simplify the interaction between Java objects and databases. ORM frameworks map Java objects to database tables, automatically generating SQL queries and handling data retrieval and persistence behind the scenes.
While databases offer powerful capabilities, they also require additional setup, maintenance, and resources. You need to install and configure a database server, design and optimize the database schema, and ensure appropriate security measures are in place to protect the data.
Choosing the right database depends on factors like data volume, performance requirements, scalability needs, and specific use cases of your application. Consider the characteristics and strengths of different database types to select the one that best suits your project requirements.
Serialization
Serialization is a mechanism in Java that allows objects to be converted into a stream of bytes for storage or transmission. It provides a convenient way to persist complex objects and transfer them between different systems or across a network.
The serialization process involves converting the state of an object into a byte stream, which can then be stored in a file, in-memory storage, or sent over a network. The reverse process, known as deserialization, converts the byte stream back into an object, allowing the state to be recreated.
In Java, serialization is supported by the java.io.Serializable
interface. By implementing this interface, a class indicates that its objects can be serialized. The serialization process is handled by the Java runtime environment, which automatically serializes the object’s state, including its non-transient fields and non-static variables.
Here’s an example of how to make a class serializable:
java
import java.io.Serializable;
class Person implements Serializable {
private String name;
private int age;
// constructor, getters, setters, etc.
}
To serialize an object, you can use classes like ObjectOutputStream
to write the object to a file or ByteArrayOutputStream
to obtain the byte stream in memory.
Here’s an example of serializing an object to a file:
java
try (ObjectOutputStream outputStream = new ObjectOutputStream(new FileOutputStream(“person.ser”))) {
Person person = new Person(“John”, 30);
outputStream.writeObject(person);
} catch (IOException e) {
// Handle exception
}
To deserialize an object, you can use classes like ObjectInputStream
to read the object from a file or ByteArrayInputStream
to obtain the byte stream from memory.
Here’s an example of deserializing an object from a file:
java
try (ObjectInputStream inputStream = new ObjectInputStream(new FileInputStream(“person.ser”))) {
Person person = (Person) inputStream.readObject();
// Use the deserialized object
} catch (IOException | ClassNotFoundException e) {
// Handle exception
}
It is important to note that the serialization process does not capture the code of the class itself; it only captures the state of the object. To ensure successful deserialization, the class definition of the serialized object must be present in the classpath.
While serialization provides a convenient way to store and transfer object state, there are a few considerations to keep in mind:
- Versioning: If the serialized object’s class changes between serialization and deserialization, it can lead to compatibility issues. To handle this, you can use techniques like serialVersionUID or externalize the serialization process using frameworks like Apache Avro or Protocol Buffers.
- Security: Serialization can be a security risk, as serialized objects can be manipulated or used to execute malicious code. It is important to exercise caution when deserializing objects from untrusted sources.
- Cross-language compatibility: Serialized objects in Java may not be compatible with objects serialized in other programming languages due to differences in serialization formats and mechanisms.
Serialization is not suitable for long-term data storage or when working with large datasets. It is primarily used for short-term persistence or for transferring object state between different components of a Java application.
Overall, serialization provides a convenient way to store and transfer object state in Java, making it a useful tool in certain scenarios. However, it’s important to consider its limitations and use it judiciously based on the specific requirements of your application.
Read more: How To Store Research Data Securely
JSON/XML
JSON (JavaScript Object Notation) and XML (eXtensible Markup Language) are popular formats for representing structured data. They provide a flexible and human-readable way to store and exchange data between different systems or components of an application.
JSON:
JSON is a lightweight data interchange format that is easy for humans to read and write and easy for machines to parse and generate. It is widely used in web applications and APIs for transmitting and storing data. JSON represents data using key-value pairs, arrays, and nested objects. It supports various data types such as numbers, strings, booleans, arrays, and objects.
In Java, you can work with JSON using libraries like Jackson, Gson, or JSON-B (part of the Java EE specification). These libraries provide APIs to serialize Java objects into JSON format and deserialize JSON into Java objects.
Here’s an example of serializing a Java object into JSON using the Jackson library:
java
import com.fasterxml.jackson.databind.ObjectMapper;
ObjectMapper objectMapper = new ObjectMapper();
Person person = new Person(“John”, 30);
String json = objectMapper.writeValueAsString(person);
The resulting JSON string will be like:
json
{
“name”: “John”,
“age”: 30
}
To deserialize the JSON string back into a Java object, you can use the following code:
java
Person person = objectMapper.readValue(json, Person.class);
XML:
XML is a markup language that allows users to define their own markup tags, making it highly flexible for representing structured data. XML uses tags to define elements, which can have attributes and contain nested elements. It is widely used in various domains, including web services, configuration files, and data interchange.
In Java, you can work with XML using libraries like JAXB (Java Architecture for XML Binding) or DOM (Document Object Model). JAXB provides a convenient way to map Java objects to XML and vice versa, allowing you to easily read and write XML data in your Java applications.
Here’s an example of using JAXB to marshal a Java object into XML:
java
import javax.xml.bind.JAXBContext;
import javax.xml.bind.Marshaller;
JAXBContext jaxbContext = JAXBContext.newInstance(Person.class);
Marshaller marshaller = jaxbContext.createMarshaller();
marshaller.setProperty(Marshaller.JAXB_FORMATTED_OUTPUT, true);
Person person = new Person(“John”, 30);
marshaller.marshal(person, System.out);
The resulting XML output will be like:
xml
To unmarshal the XML back into a Java object, you can use the following code:
java
Unmarshaller unmarshaller = jaxbContext.createUnmarshaller();
Person person = (Person) unmarshaller.unmarshal(xmlFile);
Both JSON and XML provide a flexible and readable way to store and exchange structured data. The choice between JSON and XML depends on factors like compatibility with existing systems, performance requirements, and the specific use case of your application.
It is worth noting that there are other serialization formats and libraries available in Java, such as Apache Avro and Protocol Buffers. These formats provide more compact binary representations and additional features like schema evolution and language-agnostic compatibility. Consider these options if you require more advanced serialization capabilities.
Overall, JSON and XML are valuable tools for representing structured data in a readable and flexible manner, enabling seamless communication and interoperability between different components of a Java application.
Conclusion
In this article, we have explored the various data storage options available in Java. Understanding and selecting the appropriate data storage method is essential for efficient and reliable application development.
We started by looking at the built-in data storage options provided by Java itself. Arrays offer direct access to elements and are suitable for fixed-size data, while collections like ArrayList and HashSet offer dynamic resizing and additional operations.
We then delved into external data storage options. Databases, such as MySQL and MongoDB, provide powerful querying capabilities, transaction support, data integrity enforcement, and scalability. Serialization allows objects to be converted into a stream of bytes, enabling short-term persistence and object transfer between components. JSON and XML formats offer human-readable ways to store and exchange structured data between systems and applications.
Each storage option has its own strengths and considerations. When making a choice, consider factors such as data volume, performance requirements, data structure, querying needs, and persistence requirements.
It is important to note that different storage methods can be combined to optimize data storage in Java applications. For example, you can use arrays or collections for in-memory operations and databases or files for long-term persistence. Serialization and JSON/XML formats can be used for short-term object transfer or data interchange.
As a Java developer, understanding the characteristics, advantages, and best practices of different data storage options is crucial for creating efficient, scalable, and maintainable applications.
In conclusion, the choice of data storage method in Java depends on the specific requirements of your project. By leveraging the built-in storage options and exploring external options such as databases, serialization, and JSON/XML, you can design and implement robust and effective data storage solutions in your Java applications.
Frequently Asked Questions about How To Store Data In Java
Was this page helpful?
At Storables.com, we guarantee accurate and reliable information. Our content, validated by Expert Board Contributors, is crafted following stringent Editorial Policies. We're committed to providing you with well-researched, expert-backed insights for all your informational needs.
0 thoughts on “How To Store Data In Java”