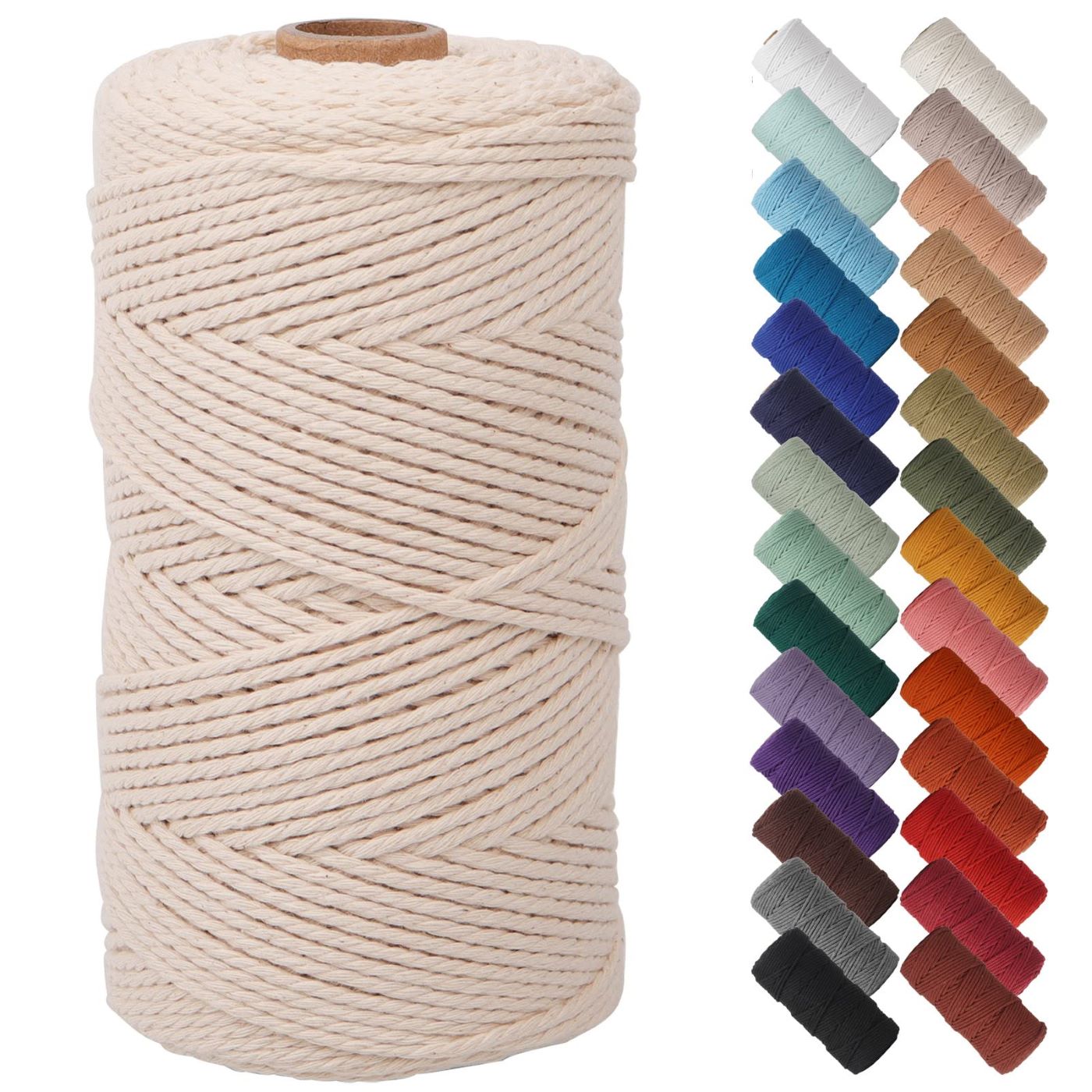
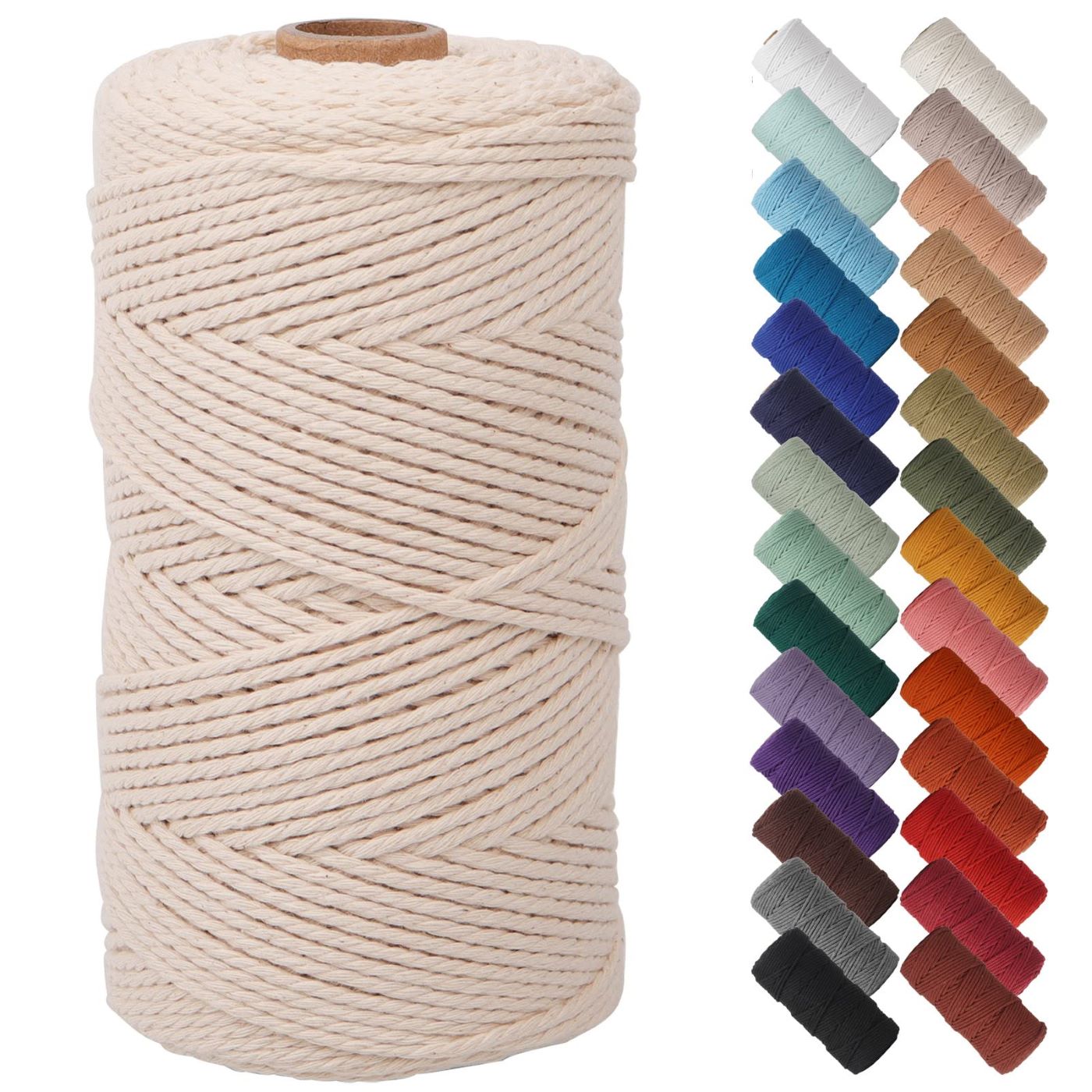
Articles
How To Store String
Modified: January 21, 2024
Learn the best way to store string articles with our step-by-step guide. Proper storage techniques ensure the longevity and quality of your strings for various projects.
(Many of the links in this article redirect to a specific reviewed product. Your purchase of these products through affiliate links helps to generate commission for Storables.com, at no extra cost. Learn more)
Introduction
A string is a fundamental data type in computer programming languages that represents a sequence of characters. Strings are used to store and manipulate text-based data such as names, addresses, and messages. Understanding how to store strings efficiently is crucial for optimizing memory usage and improving the performance of applications.
In this article, we will explore various techniques for storing strings and discuss their advantages and disadvantages. Choosing the right string storage technique can have a significant impact on the efficiency and speed of your program, so it’s essential to understand the different options available.
By the end of this article, you will have a better understanding of the importance of string storage and will be able to make an informed decision when it comes to selecting the appropriate storage technique for your specific use case.
Key Takeaways:
- Efficient string storage is crucial for optimizing memory usage and improving application performance. Understanding the various storage techniques and their trade-offs is essential for making informed decisions.
- The choice of string storage technique depends on factors such as string length, performance needs, memory constraints, data integrity, and language/library support. No single technique is ideal for all situations, and a combination of techniques may be necessary.
Read more: How To Store String Lights
Understanding Strings
Before delving into the intricacies of string storage, it’s important to have a solid understanding of what strings are and how they are represented in computer memory.
In most programming languages, a string is simply a sequence of characters. Each character is typically represented using a specific encoding system, such as ASCII or Unicode. This encoding system maps each character to a unique numeric value, allowing the computer to store and manipulate strings.
Strings can be of varying lengths, from just a single character to hundreds or even thousands of characters. When a string is stored in memory, it is typically allocated a continuous block of memory to hold all the characters. This block of memory is commonly referred to as a string buffer or string object.
One important aspect to note is that strings are often immutable, meaning that once a string is created, its contents cannot be changed. Instead, any operation that appears to modify a string actually creates a new string with the desired changes. This immutability property has significant implications for string storage techniques.
Now that we have a basic understanding of strings and how they are represented, let’s explore the importance of efficient string storage and the various techniques that can be used.
Importance of String Storage
Efficient string storage is vital for optimizing memory usage and improving the performance of an application. Strings can consume a significant amount of memory, especially when dealing with large datasets or processing large volumes of text-based data.
Here are some key reasons why string storage is important:
- Memory Usage: Strings can take up a considerable amount of memory, especially in scenarios where multiple strings need to be stored simultaneously. Efficient string storage techniques can help reduce memory usage and prevent memory-related issues, such as running out of memory.
- Performance: String operations, such as concatenation, searching, and manipulation, can be resource-intensive. The chosen string storage technique can greatly impact the performance of these operations. By using an optimal storage technique, you can enhance the speed and efficiency of string-related operations.
- Scalability: As data volumes grow, the efficient storage of strings becomes even more critical. Without proper string storage techniques, the size and complexity of string-based operations can increase exponentially, leading to degraded performance and scalability issues.
- Resource Utilization: Efficient storage of strings ensures optimal utilization of system resources, such as memory and processing power. It allows for the effective management of limited resources in resource-constrained environments.
- Data Integrity: Improper string storage techniques can result in data corruption or loss. It is crucial to choose a storage method that maintains the integrity of the string data and prevents any unintended modifications.
By understanding the importance of string storage, we can now explore various common techniques used for storing strings efficiently. Each technique has its own advantages and disadvantages, making it essential to choose the appropriate technique based on the specific requirements of your application.
Common String Storage Techniques
There are several commonly used techniques for storing strings efficiently. Each technique has its own characteristics, advantages, and trade-offs. Let’s explore some of the most common string storage techniques:
- Inline Storage: In this technique, the string is stored directly within a fixed-size data structure or variable. It is suitable for storing short strings with known lengths. Inline storage eliminates the need for dynamic memory allocation and offers fast access to the string. However, it may limit the maximum length of the string and can lead to wastage of memory if the allocated space is not fully utilized.
- Array Storage: This technique involves storing strings in an array of characters. Each character occupies a fixed amount of memory, and consecutive characters form the string. Arrays provide efficient random access to characters, making it easy to retrieve or modify specific positions within the string. However, they have fixed lengths and can be wasteful if the string length is variable or unknown.
- Linked List Storage: Linked lists are a dynamic data structure where each node contains a character and a reference to the next node. This technique can efficiently handle strings of any length and allows for easy insertion and deletion of characters. However, random access to characters becomes less efficient as it requires traversing the list from the beginning.
- Hash Table Storage: Hash tables can be used to store strings as key-value pairs. The string is hashed to generate an index, which is used to store and retrieve the string efficiently. Hash tables offer fast retrieval and insertion operations, making them suitable for scenarios where quick access to string data is essential. However, they may consume more memory due to the overhead of hash table structures.
Each of these string storage techniques has its own set of advantages and considerations. The choice of technique depends on factors such as the expected length of strings, the frequency and types of operations performed on the strings, and the available system resources. It’s important to carefully evaluate these factors and choose the appropriate storage technique for your specific use case.
Inline Storage
Inline storage is a string storage technique where the string is stored directly within a fixed-size data structure or variable. It is suitable for storing short strings with known lengths. In this technique, the memory for the string is allocated statically and is part of the same data structure or variable that holds other related data.
One of the main advantages of inline storage is its fast access time. Since the string is stored directly within the data structure, retrieval and modification of the string can be done with minimal overhead. This makes it an efficient storage technique for small strings that need to be accessed frequently.
Another benefit of inline storage is that it eliminates the need for dynamic memory allocation. Since the memory for the string is allocated statically, there is no need to allocate or deallocate memory during runtime. This can simplify memory management and reduce the overhead associated with dynamic memory allocation.
However, inline storage has some limitations. The maximum length of the string is determined by the size of the data structure or variable that holds the string. If the string exceeds this size, it may result in truncation or loss of data. Additionally, if the allocated space is not fully utilized, it can lead to wastage of memory.
Inline storage is commonly used in programming languages that provide built-in data types for handling strings, such as C-style strings in C and C++, or strings in languages like Python and Java. In such cases, the size of the string is typically pre-determined based on the maximum expected length of the string.
Overall, inline storage is a suitable choice for storing short strings with fixed lengths. It provides fast access to the string data and eliminates the need for dynamic memory allocation. However, it is important to carefully consider the maximum length and memory utilization to avoid potential issues with truncation or wasted memory.
Store strings in a dry, cool place away from direct sunlight and moisture to prevent them from becoming brittle or discolored. Consider using acid-free tissue paper or archival boxes for long-term storage.
Read more: How To Store String Beans
Array Storage
Array storage is a common technique for storing strings where the characters of the string are stored in an array. Each character occupies a fixed amount of memory, and consecutive characters form the string. Array storage is suitable for strings of varying lengths, even those with unknown lengths at compile-time.
One of the main advantages of array storage is its efficient random access to characters. Since the characters are stored in a contiguous block of memory, accessing a specific character by its index can be done in constant time. This makes it easy to retrieve or modify individual characters within the string, which is useful in many string operations.
Array storage also allows for efficient iteration over the characters of the string. By sequentially accessing each element of the array, you can process the string in a linear manner.
However, array storage has some limitations. The size of the array must be determined in advance, which can lead to wastage of memory if the string length is variable or unknown. If the array is not large enough to accommodate a string, it may result in truncation or loss of data.
Another consideration is that arrays are allocated statically, meaning that the memory space for the string is allocated at compile-time or initialization. This makes it less flexible in scenarios where the size of the string may change dynamically during runtime. In such cases, the array may need to be resized or a new array allocated, which incurs additional overhead.
Array storage is commonly used in programming languages where strings are represented as an array of characters, such as C and C++. In these languages, a null character (‘